React State Management & Clean SPA Architecture
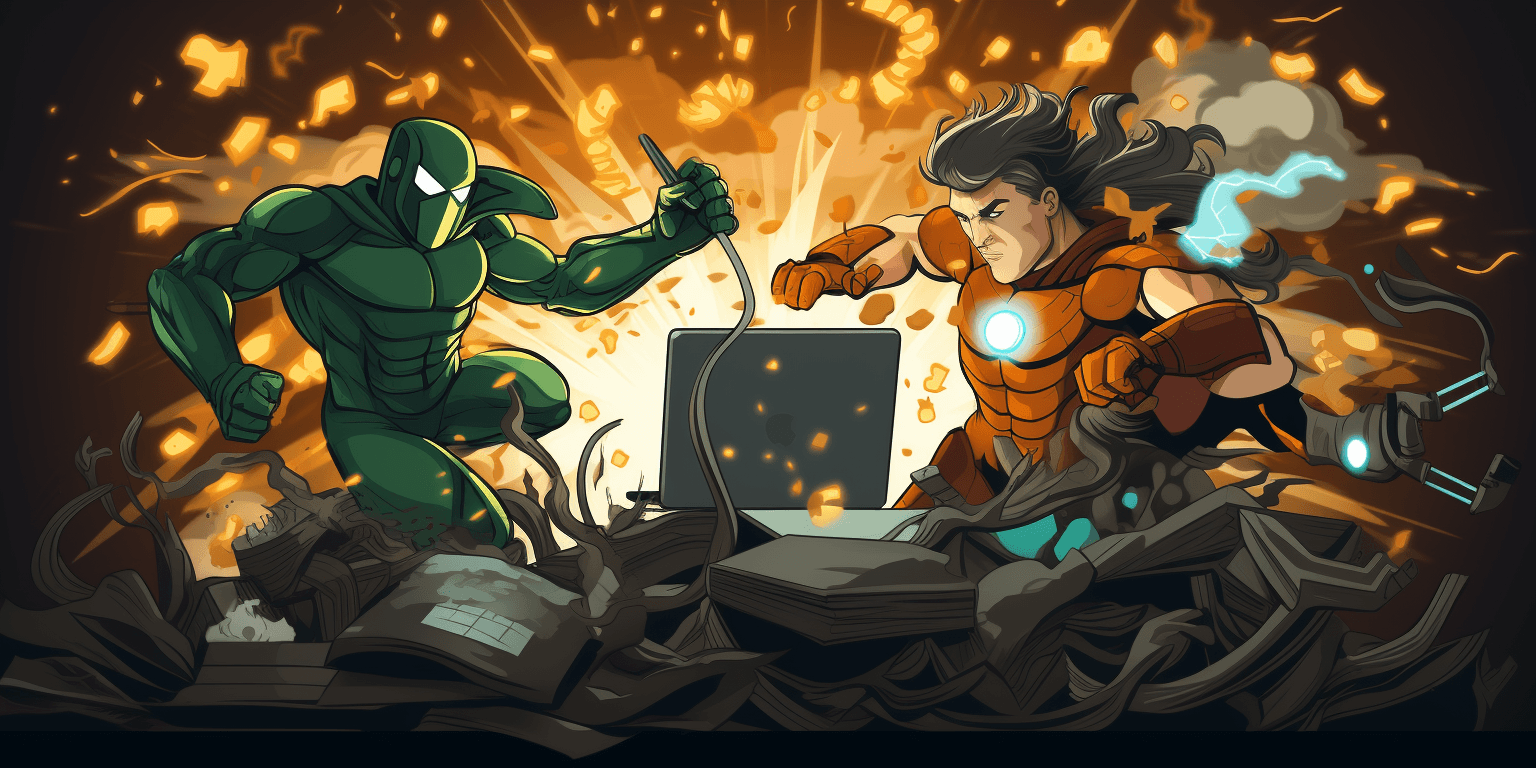
React state management approaches and their relationships
When Does State Management Matter?
With the rise of Server-Side Rendering (SSR) frameworks like Next.js, traditional client-side state management has become less critical for many applications. However, understanding React state management remains essential for several reasons:
- Many existing applications are built as Single Page Applications (SPAs)
- Complex interactive UIs still require sophisticated client-side state
- Certain features demand real-time state synchronization across components
This article explores the most popular state management solutions in the React ecosystem: Redux, Zustand, Recoil, and the Context API. We'll examine their strengths, weaknesses, and ideal use cases to help you make informed decisions for your projects.
The Prop Drilling Problem
Before diving into dedicated state management libraries, let's understand the problem they solve. In React's component-based architecture, data flows from parent to child via props. This approach, while straightforward, can lead to what's known as "prop drilling."
What is Prop Drilling?
Prop drilling occurs when props need to be passed through multiple layers of components that don't actually use those props, but merely pass them down to deeper components.
Prop drilling has several limitations:
- Limited to Parent-Child Relationships: It works well for direct parent-child communication but becomes unwieldy in deeply nested component trees
- Maintenance Complexity: As your application grows, passing props through numerous intermediate components creates maintenance challenges
- Readability Issues: Components become cluttered with props they only pass through but don't actually use
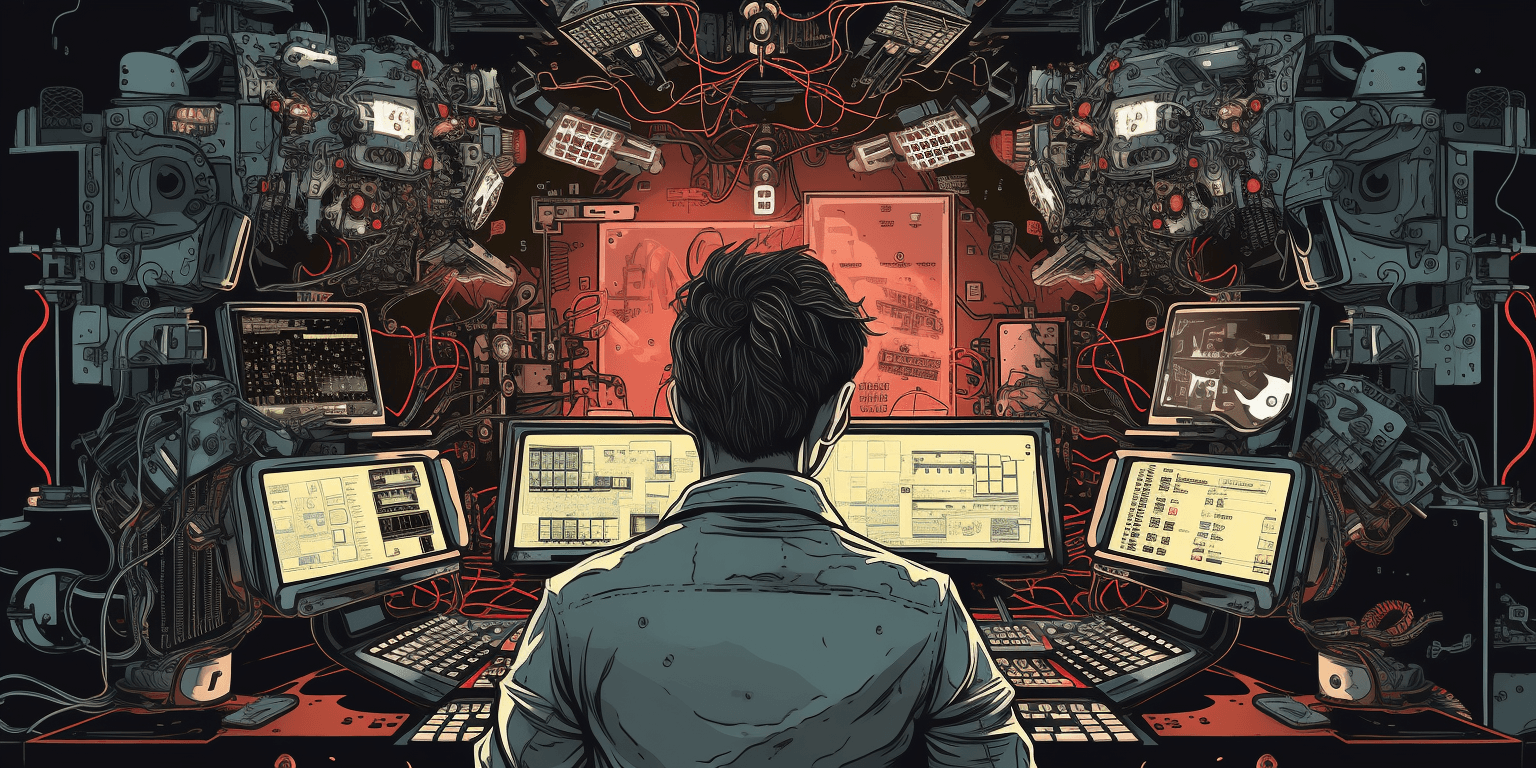
Prop drilling versus centralized state management
Understanding State Management Fundamentals
Before comparing specific libraries, let's establish a clear understanding of state management concepts in React.
Local vs. Global State
React state exists in two primary forms:
Type | Scope | Typical Use Cases | Implementation |
---|---|---|---|
Local State | Single component | Form inputs, UI toggles, component-specific data | useState , useReducer |
Global State | Multiple components | User authentication, theme settings, shopping cart | Redux, Context API, etc. |
Core State Management Principles
Regardless of which tool you choose, several principles remain consistent across state management solutions:
-
Immutability: State should never be directly modified. Instead, create new state objects that replace the previous state.
-
Single Source of Truth: Maintaining one authoritative location for each piece of state helps prevent synchronization issues.
-
Unidirectional Data Flow: Data should flow in one direction, making the application's behavior more predictable.
-
Actions and Reducers: Many state management libraries use the concept of dispatching actions that are processed by reducers to update state.
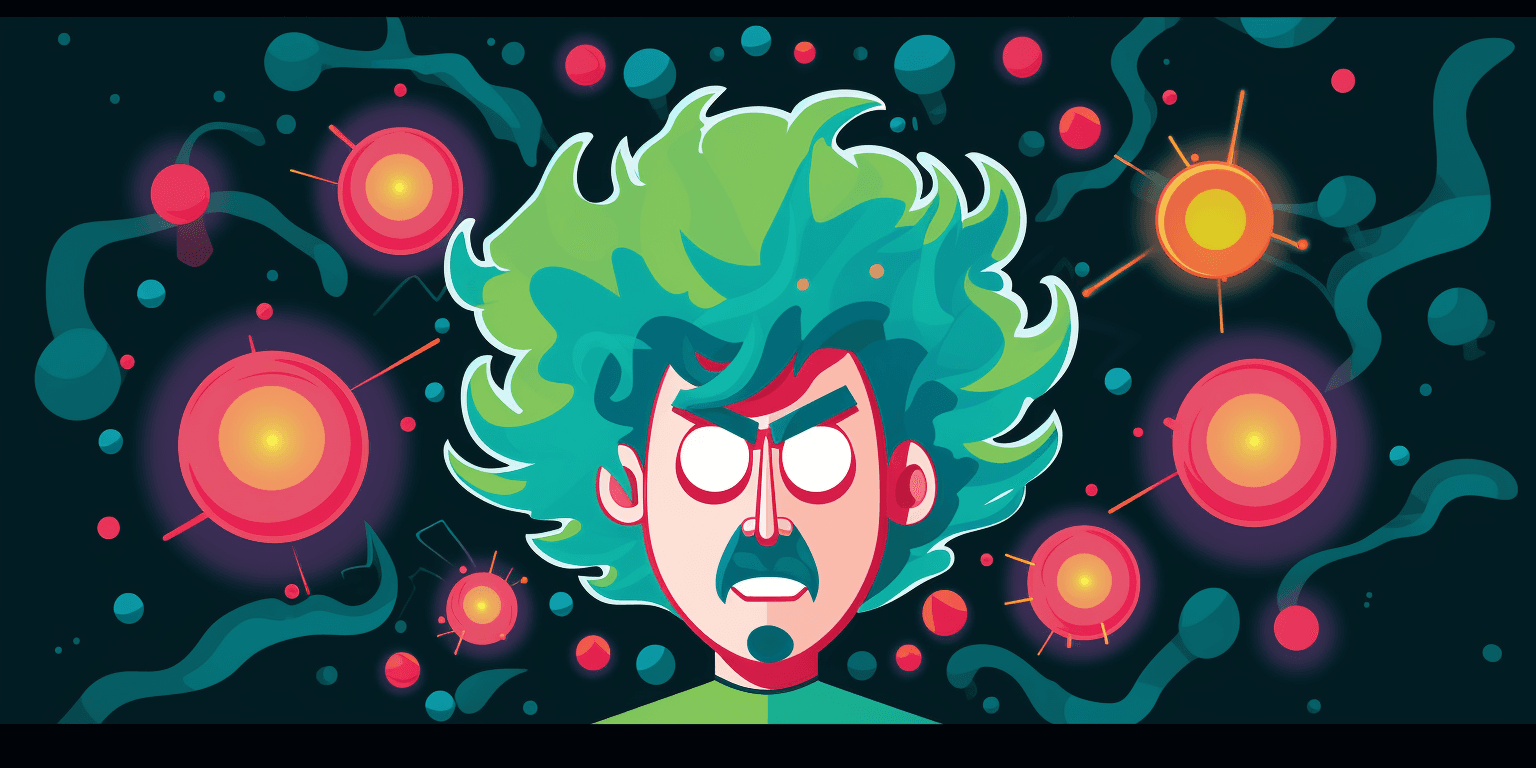
Unidirectional data flow in modern React state management
Redux: The Industry Standard
Redux has long been the go-to state management solution for React applications with complex state requirements. It implements a predictable state container based on three key principles:
- The entire application state is stored in a single store
- State can only be changed by dispatching actions
- Reducers specify how actions transform the current state into the next state
When to Choose Redux
// Example Redux implementation
import { createStore } from 'redux';
// Reducer
function counterReducer(state = { count: 0 }, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
}
// Store
const store = createStore(counterReducer);
// Dispatching actions
store.dispatch({ type: 'INCREMENT' });
Redux excels in several scenarios:
- Large-scale applications with complex state interdependencies
- Projects requiring extensive debugging capabilities (time-travel debugging)
- Teams that value strict patterns and conventions
- Applications needing middleware support for side effects (Redux Thunk, Redux Saga)
Redux Pros and Cons
Advantages
- Mature, battle-tested ecosystem
- Excellent DevTools for debugging
- Predictable state updates
- Extensive middleware support
- Large community and resources
Disadvantages
- Significant boilerplate code
- Steeper learning curve
- Can be overkill for smaller applications
- More complex setup process
- Larger bundle size
Zustand: Simplicity and Performance
Zustand has gained rapid adoption by offering a minimalist approach to state management. It provides a hook-based API that feels natural in the React ecosystem while avoiding the verbosity of Redux.
When to Choose Zustand
// Example Zustand implementation
import create from 'zustand';
const useStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
// Using in a component
function Counter() {
const { count, increment, decrement } = useStore();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
Zustand is particularly well-suited for:
- Modern React applications using hooks extensively
- Projects prioritizing developer experience and simplicity
- Applications where performance and bundle size are critical
- Teams looking for a minimal learning curve
Zustand Pros and Cons
Advantages
- Extremely simple API
- Tiny bundle size (~1KB)
- No provider components needed
- Works with React Concurrent Mode
- TypeScript support out of the box
Disadvantages
- Less established ecosystem
- Fewer middleware options
- Limited debugging capabilities
- Less guidance for larger applications
- Smaller community compared to Redux
Recoil: Facebook's Approach to React State
Recoil was developed by Facebook specifically to address React's state management needs. It introduces a unique atom-based approach that works particularly well with React's component model.
When to Choose Recoil
// Example Recoil implementation
import { atom, useRecoilState, selector, useRecoilValue } from 'recoil';
// Define an atom (a piece of state)
const countState = atom({
key: 'countState', // unique ID
default: 0, // default value
});
// Define a selector (derived state)
const doubleCountState = selector({
key: 'doubleCountState',
get: ({get}) => {
return get(countState) * 2;
},
});
// Using in a component
function Counter() {
const [count, setCount] = useRecoilState(countState);
const doubleCount = useRecoilValue(doubleCountState);
return (
<div>
<p>Count: {count}</p>
<p>Double Count: {doubleCount}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Recoil is ideal for:
- Applications with complex derived state requirements
- Teams already heavily invested in the React ecosystem
- Projects needing fine-grained reactivity control
- Use cases involving asynchronous state dependencies
Recoil Pros and Cons
Advantages
- Excellent derived state capabilities
- Built for React's component model
- Strong TypeScript integration
- Supports React Concurrent Mode
- Powerful async selectors
Disadvantages
- Still in experimental phase
- Smaller community and resources
- API may change in future releases
- More complexity than Zustand
- Requires React-specific knowledge
Context API: React's Built-in Solution
The Context API is React's native solution for passing data through the component tree without manually passing props at every level. It's not a complete state management solution but provides the foundation for simpler state management patterns.
When to Choose Context API
// Example Context API implementation
import React, { createContext, useContext, useState } from 'react';
// Create a context
const CountContext = createContext();
// Provider component
function CountProvider({ children }) {
const [count, setCount] = useState(0);
return (
<CountContext.Provider value={{ count, setCount }}>
{children}
</CountContext.Provider>
);
}
// Consumer component
function Counter() {
const { count, setCount } = useContext(CountContext);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
// Usage
function App() {
return (
<CountProvider>
<Counter />
</CountProvider>
);
}
The Context API is best suited for:
- Smaller applications with modest state needs
- Projects where you want to avoid external dependencies
- Teams looking for a lightweight, built-in solution
- Applications with well-defined, isolated state domains
Context API Pros and Cons
Advantages
- Built into React - no dependencies
- Simple mental model
- No additional bundle size
- Perfect for theme/auth state
- Works seamlessly with hooks
Disadvantages
- Performance concerns with large state
- No built-in memoization
- Can lead to render inefficiency
- No time-travel debugging
- Nested providers become unwieldy
Making the Right Choice for Your Project
Selecting the appropriate state management solution depends on several factors:
Deciding Factors
-
Application Scale: Larger applications typically benefit from more structured approaches like Redux, while smaller applications might be better served by Context API or Zustand.
-
Team Experience: Consider your team's familiarity with different solutions. Redux has a steeper learning curve but is widely known.
-
Performance Requirements: If performance is critical, Zustand's lightweight approach or Recoil's fine-grained updates might be preferable.
-
Future Scalability: Even if your application starts small, consider how it might grow and whether your state management solution can scale accordingly.
Decision Framework
- Choose Redux if you need a proven solution with extensive middleware support for complex applications.
- Choose Zustand if you want simplicity, minimal boilerplate, and excellent performance.
- Choose Recoil if you need sophisticated derived state capabilities and are building a React-focused application.
- Choose Context API if you're building smaller applications and want to avoid external dependencies.
Conclusion
As React continues to evolve, so do the approaches to state management. There is no one-size-fits-all solution, and the "best" choice depends entirely on your specific project requirements, team expertise, and architectural preferences.
Whether you choose the robust ecosystem of Redux, the simplicity of Zustand, the React-native approach of Recoil, or the built-in Context API, understanding the core principles of state management will help you build more maintainable and performant React applications.
Remember that you can also mix approaches—using Context for theme settings while implementing Redux for complex business logic, for instance. The key is selecting the right tool for each specific state management need in your application.
What's your preferred state management solution? Have you had success with approaches not covered here? The React ecosystem continues to evolve, and sharing experiences helps everyone make better architectural decisions.